When it comes to NoSQL databases, MongoDB is frequently cited as one of the best options. NoSQL databases, as opposed to traditional SQL databases, are known for their ability to work with large datasets, providing scalability and flexibility in app development. SQL databases, on the other hand, are relatively stiff and difficult to scale when dealing with large data sets.
In this article, we’ll look at 5 easy steps for the Angular MongoDB Database Integration.
Table of Contents
Steps to Get Started with Angular MongoDB Integration
A stable and dependable MongoDB connection is essential when using Angular to construct a web application. This entails setting up the Angular MongoDB connection to provide smooth communication between the back-end MongoDB database and the front-end Angular framework for efficient data retrieval and manipulation. Take a look at the steps below to learn how to connect Angular with MongoDB.
- Angular MongoDB Integration Step 1: Using the Angular CLI, Create a basic Angular 5 App
- Angular MongoDB Integration Step 2: Let’s set up Express, Mongoose, and the body-parser
- Angular MongoDB Integration Step 3: Make a new file called server.js
- Angular MongoDB Integration Step 4: Create a new Angular Service to call common AJAX APIs
- Angular MongoDB Integration Step 5: It’s time to put our app to use!
Hevo is the only real-time ELT No-code Data Pipeline platform that cost-effectively automates data pipelines that are flexible to your needs. With integration with 150+ Data Sources (60+ free sources), we help you not only export data from sources & load data to the destinations but also transform & enrich your data, & make it analysis-ready.
Check out what makes Hevo amazing:
- It has a highly interactive UI, which is easy to use.
- It streamlines your data integration task and allows you to scale horizontally.
- The Hevo team is available around the clock to provide exceptional support to you.
With its automated data handling and no-code interface, Hevo makes building efficient pipelines effortless.
Get Started with Hevo for FreeStep 1: Using the Angular CLI, Create a basic Angular 5 App
Install the Angular CLI in the terminal.
sudo npm install -g @angular/cli
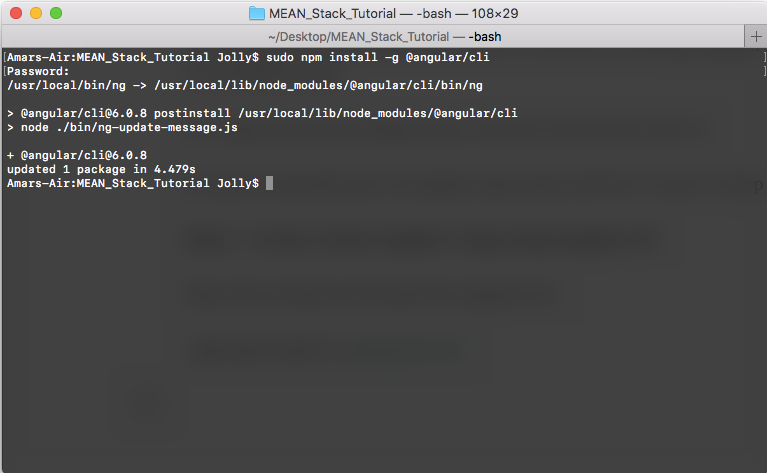
So far, we’ve installed the AngularJS Command Line Interface. It’s time to start working on the new Angular App.
Enter the following command:
ng new <name-of-the-app>
Now let’s change the directory to the directory of our app.
cd <name-of-the-app>
It’s time to put our Angular App to the test!
ng serve -o
Our browser displays the following page:
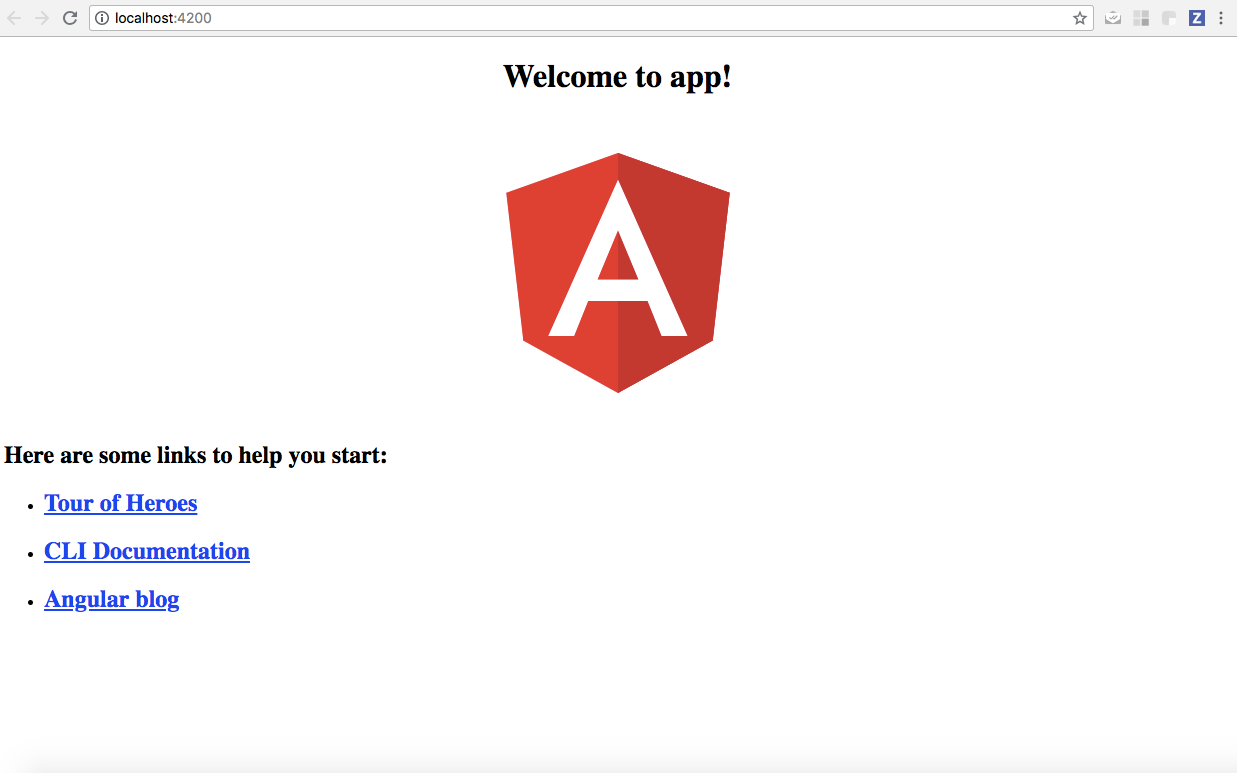
Step 2: Let’s set up Express, Mongoose, and the body-parser
npm install express — save
npm install mongoose — save
npm install body-parser --save
- These commands install specific Node.js packages using npm (Node Package Manager) for your project.
npm install express --save
installs the Express framework, which is used for building web applications and APIs.npm install mongoose --save
installs Mongoose, a library for MongoDB that simplifies database interactions.npm install body-parser --save
installs Body-Parser, middleware for parsing incoming request bodies in a middleware before your handlers.- The
--save
flag adds these packages to your project’spackage.json
file, ensuring they are listed as dependencies.
Express is a web framework for routing and middleware that has very little functionality on its own and is essentially a series of middleware function calls.
In the application’s request-response cycle, middleware functions can access the request object (req), the response object (res), and the subsequent middleware function. To indicate the next middleware function, a variable named next is frequently used.
Mongoose facilitates the connection between the NodeJS app and the MongoDB database.
The Body-Parser middleware facilitates the parsing of incoming request bodies in a middleware prior to the execution of handlers that are accessed through the req.body property.
Step 3: Make a new file called server.js
In the text editor, open the server.js file.
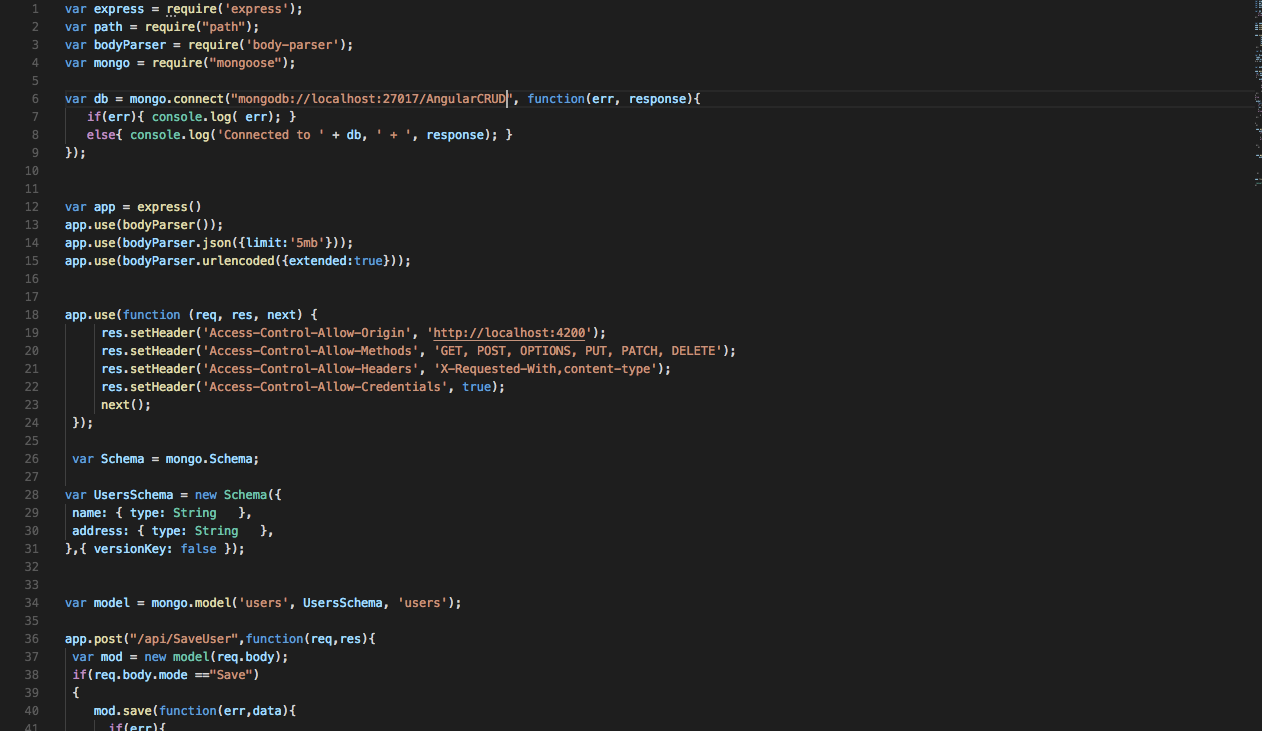
We may utilize the packages we imported, as stated in lines 1-4. Lines 5–9 create a local instance of a MongoDB database. If a problem arises and the database is unable to establish a connection, we notify the user of the error. The headers are defined at lines 18–24.
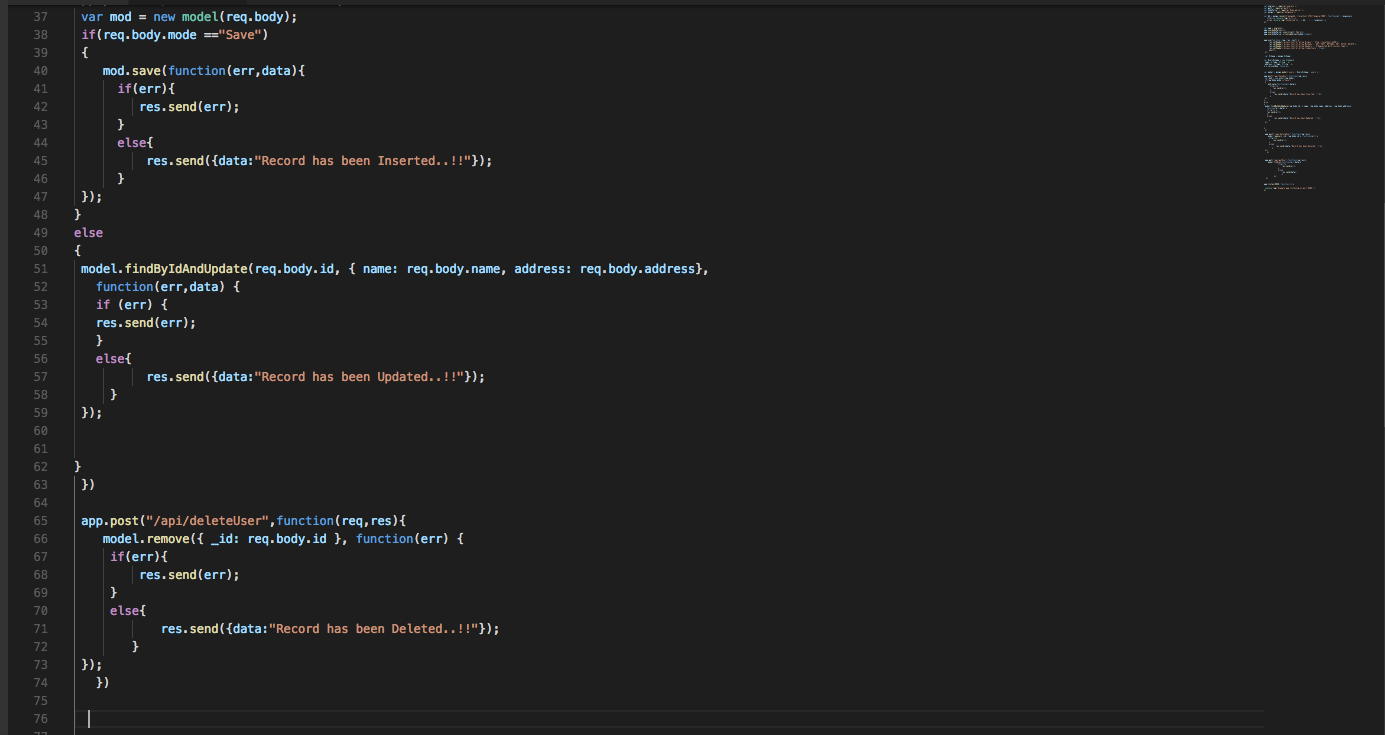
The lines 26–31 help us define the structure of our database. We will just save the user’s name and address in our example, as they are both Strings. Lines 36–87 include the definitions of our API calls, which enable us get, store, edit, and remove user data from the database.
Step 4: Create a new Angular Service to call common AJAX APIs
Create a new Angular Service to call common AJAX APIs.
ng g s common -spec=false
- This command is used in Angular to generate a new service named
common
. - The
ng
stands for Angular CLI (Command Line Interface), which helps automate tasks in Angular development. - The
g
is a shorthand forgenerate
, indicating that you want to create a new file. - The
-spec=false
option disables the generation of a spec file, which is typically used for testing. - As a result, this command creates a new service without the accompanying test file, streamlining the process for developers who may not need tests immediately.
Open src/app/common.service.ts and paste the following code there:

Finally, we update our app’s HTML to display the data!
Step 5: It’s time to put our app to use!
- Use mongod to launch a local instance of MongoDB.
- A couple of similar-looking commands appear on the terminal, and the pointer remains there, waiting for connections to the MongoDB Daemon to be established.
- Now, let’s try running our Angular App with the ng serve -o command.

Conclusion
You’ve learned how to create a basic CRUD application using Angular 5, Nodejs, Express, and the Angular MongoDB Integration.
To meet the growing storage and computing needs of data, you will need to invest some of your engineering bandwidth in integrating data from all sources, cleaning and transforming it, and finally loading it into a Cloud Data Warehouse for further Business Analytics. A Cloud-Based ETL tool, such as Hevo Data, can efficiently handle all of these challenges. Try a 14-day free trial and experience the feature-rich Hevo suite firsthand. Also, check out our unbeatable pricing to choose the best plan for your organization.
FAQ on Angular MongoDB Integration
Can I use MongoDB with Angular?
Yes, you can use MongoDB with Angular by creating a backend with Node.js that interfaces with MongoDB and serves data to your Angular application via RESTful APIs or GraphQL.
Can Angular directly connect to a database?
No, Angular cannot directly connect to a database. You need a backend service (like Node.js, Django, or Flask) to handle database operations and provide an API with which Angular can interact.
How to store data in MongoDB using Angular?
Create a backend service with Node.js and Express to store data in MongoDB using Angular. Use Mongoose to interact with MongoDB and create API endpoints that Angular can call to perform CRUD operations.
Can we use MySQL with Angular?
Yes, you can use MySQL with Angular by setting up a backend server (like Node.js, Django, or Flask) that connects to MySQL and serves data to Angular through RESTful APIs.
How to use MongoDB with NPM?
Install the MongoDB Node.js driver with npm install mongodb. Use it in your Node.js application to connect to MongoDB, perform CRUD operations, and handle database interactions.