Organizations continuously update their applications and services to keep them up to date with the technology. Modern applications stream data using APIs while delivering smooth performance. Large websites and applications fetch huge volumes of data and to get exactly what data they need is a complex task.
GraphQL is a language designed for handling APIs requests that enable users to query, access, and manipulate data through an intuitive and flexible syntax. AWS Lambda is a serverless technology that makes it easier for users to run code in response to events. AWS Lambda GraphQL Integration helps Developers manage thousands of files and business logic.
It allows you to request only the data that is needed and a runtime for fulfilling those queries with your existing data. In this article, you will learn about the steps to set up AWS Lambda GraphQL Integration.
Table of Contents
What is AWS Lambda?
AWS Lambda is an event-driven, serverless computing service provided by Amazon Web Services that lets users run their code without provisioning and managing any infrastructure or servers. AWS Lambda runs the code in high availability compute infrastructure and manages all computing resources administration, maintenance, scaling, monitoring, and logging. Lambda allows users to run any type of application and service provided the code is in the language that Lambda supports.
Key Features of AWS Lambda
AWS Lambda is an excellent tool when there is a requirement for serverless computing. A few key features of AWS Lambda are listed below:
- Functions Defined as Container Images: AWS Lambda allows users to user-preferred container image tooling, workflows, and dependencies to build, test, and deploy Lambda functions.
- Lambda Extensions: You can use Lambda extensions to augment your Lambda functions. For example, use extensions to integrate Lambda with your favorite tools for monitoring, observability, security, and governance.
- Function Blueprints: AWS Lambda provides function blueprints that provide sample code to use AWS Lambda with other third-party applications. Users can easily use the blueprints to customize and deploy their application in AWS Lambda.
- Database Access: AWS Lambda manages a pool of database connections and relays queries from a function. It allows functions to reach high concurrency levels without disturbing Database connections.
- File Systems Access: AWS Lambda supports file system access through mounting. It allows users to mount Amazon Elastic File System to access the content securely and at high concurrency.
Hevo, an enterprise-grade data pipeline as a service, enables real-time data transfer from various sources to any destination without requiring any coding. Designed to handle large-scale data, Hevo ensures that your data is transferred reliably, accurately, and consistently. Key Features of Hevo:
- Fully Managed: Hevo is a fully automated platform, eliminating the need for management and maintenance.
- Easy to Use: With its simple and interactive UI, Hevo is user-friendly, making it easy for new customers to perform operations.
- Schema Management: Hevo automatically detects the schema of incoming data and maps it to the destination schema.
Simplify your data warehousing and analysis with Hevo today!
Get Started with Hevo for FreeWhat is GraphQL?
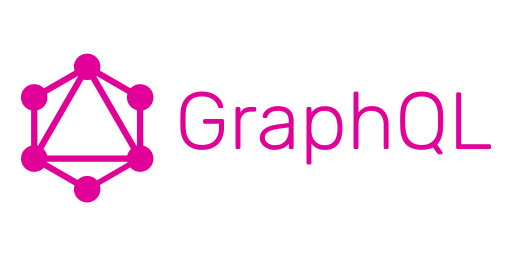
GraphQL is a query language built for APIs to query the existing data. GraphQL provides a complete description of API data that provides flexibility to ask for exactly what the client needs and nothing more, making it easier to evolve APIs over time and enabling powerful developer tools.
GraphQL isn’t tied to any specific database and storage engine, and hence it can query data from any Database as it is backed by existing code and the data.
Once the GraphQL server is up and running, users can submit the queries on the server, validating the query and executing them. The GraphQL server first checks a query to ensure it only refers to the types and fields defined and then runs the provided functions to produce a result.
For example, a GraphQL query like the below:
{
me {
name
}
}
Could produce the following JSON result:
{
"me": {
"name": "Luke Skywalker"
}
}
Key Features of GraphQL
- Fetching Data with a Single API Call: GraphQL allows users to retrieve the data from a single API call instead of the REST endpoint, which has individual endpoints to retrieve the data.
- No over and Under-Fetching Problems: GraphQL knows what precisely the client is requesting, and hence the response contains the exact data in the single request as opposed to REST responses, which are known for either containing too much data or not enough of it.
- Detailed Error Messages: GraphQL provides detailed error messages that include all the resolvers and faulty parts of the queries.
- API Evolution: GraphQL creates a uniform API across the entire application that solves the problem of API versioning and API evolution. Hence, the user can remove the deprecated fields later without impacting the schema and existing queries. GraphQL APIs give apps continuous access to new features and encourage cleaner, more maintainable server code by using a single, evolving version.
Prerequisites
Now that you have a basic understanding of AWS Lambda and GraphQL, it’s time to see how you can easily deploy a GraphQL server on AWS Lambda. But before proceeding with the AWS Lambda GraphQL Integration section, let’s have a look at the pre-requisites required listed below:
- AWS account with access to IAM and Lambda.
- Serverless installed on your machine.
- Node.js v8.x or later.
- git v2.14.1 or later.
Steps for AWS Lambda GraphQL Integration
In this section, you will go through the steps to set up AWS Lambda GraphQL Integration in which you will build a GraphQL Server and deploy it on AWS Lambda. The following steps for Integration are listed below:
Step 1: Building the GraphQL Server
Open the CLI and run the below commands to download and install some packages for AWS Lambda GraphQL Integration.
mkdir serverless-orders-api && cd serverless-orders-api
git init
npm init -y
npm install apollo-server-lambda
npm install -D serverless-offline
Serverless-offline will help us to develop and test the application locally. The following steps to build the GraphQL server are listed below.
- Set Up GraphQL Handler
- Set Up Schema
- Set Up Resolvers
- Set Up the serverless.yml File
- Testing Locally
1. Setup the GraphQL Handler
Open the above-created project on any source code editor and look for the file named handler.js to modify it. Update the file as shown below to add resolvers and schema to the file to create a new Apollo server.
const { ApolloServer } = require('apollo-server-lambda');
const { resolvers } = require('./graphql/resolvers');
const { typeDefs } = require('./graphql/schema');
const server = new ApolloServer({
typeDefs,
resolvers,
});
module.exports.graphqlHandler = server.createHandler({
cors: {
origin: '*',
credentials: true,
},
});
2. Setup the Schema
In this particular use case, we will be using the hardcoded value retrieved via GraphQL(as we are not using any database to link).
The schema file defines how our data looks and its type. Create a file named schema.js inside a new folder named graphql in the project’s root and paste the following content on it.
const { gql } = require('apollo-server-lambda');
module.exports.typeDefs = gql`
type Orders {
id: String!
amount: Float!
tax: Float!
total: Float!
}
type Query {
getOrders: [Orders]
};
3. Setup the Resolvers
Resolvers are the files that provide instructions to GraphQL about queries and how to return data. Create a resolver.js file under the graphql folder and paste the below content. This is a step to put database details to fetch data, but we will return some static data in this tutorial.
module.exports.resolvers = {
Query: {
getOrders: (_, args) => {
const orders = [
{
id: '1977',
amount: 10.0,
tax: 0.5,
total: 10.5,
},
{
id: '1978',
amount: 20.0,
tax: 1.0,
total: 21.0,
},
{
id: '1979',
amount: 30.0,
tax: 1.5,
total: 31.5,
},
];
return orders;
},
},
};
4. Setup the severless.yml File
From the serverless-offline, modify the serverless.yml file and paste the following content into it.
service: serverless-graphql
plugins:
- serverless-offline
provider:
name: aws
runtime: nodejs12.x
stage: ${opt:stage, 'dev'}
region: us-east-1
memorySize: 128
functions:
graphql:
handler: handler.graphqlHandler
events:
- http:
path: graphql
method: ANY
cors: true
5. Testing Locally
Now that we have everything in place, let’s run the GraphQL server locally. Go to the root directory of the project and there tun the following command given below.
serverless offline
Open postman or any API testing tool and use the url – http://localhost:3000/dev/graphql to test the query for AWS Lambda GraphQL Integration.
Step 2: Deploying to AWS
Once the local query is tested successfully, we can deploy AWS Lambda GraphQL. To deploy GraphQL on AWS, you need to have an active AWS account with sufficient privileges to access IAM and Lambda.
Deploying AWS Lambda GraphQL is a pretty straightforward process. On the console, run the below command to deploy to AWS –
serverless deploy
Once the AWS Lambda GraphQL deployment is successful, let’s test the newly created AWS endpoint in Postman. You can provide the following POST request body, and the response of the AWS Lambda GraphQL API call will have the data associated with it. You will get the endpoint details in the success message in the terminal.
query {
orders {
id
amount
tax
total
}
}
That’s it! You have completed the AWS Lambda GraphQL Integration in which you built a GraphQL server and deployed it using AWS Lambda.
Conclusion
In this article, you learnt about AWS Lambda, GraphQL, and how to build a GraphQL server locally and then deploy it to AWS Lambda using AWS serverless, which is a pretty straightforward task. You went through the detailed steps for AWS Lambda GraphQL Integration using an example. AWS Lambda is a widely used serverless technology and it is easy to use. For seamless data integration with AWS services, explore Hevo, a no-code platform that simplifies data workflows and connects various sources with ease.
SIGN UP for a 14-day free trial and see the difference!
Share your experience of learning about running AWS Lambda GraphQL Integration in the comments section below!
FAQs
1. How to use GraphQL in AWS Lambda?
Use AWS Lambda with API Gateway to create a GraphQL server. Set up the GraphQL schema, write Lambda functions to handle queries, and integrate with services like DynamoDB or RDS. API Gateway routes GraphQL requests to the corresponding Lambda function.
2. What is the difference between Lambda and GraphQL?
AWS Lambda is a serverless computing service, while GraphQL is a query language for APIs. Lambda executes code in response to events, whereas GraphQL defines how data can be queried from a server.
3. Does AWS use GraphQL?
Yes, AWS supports GraphQL through AWS AppSync, a managed service that simplifies building GraphQL APIs by integrating with AWS services like DynamoDB, Lambda, and Elasticsearch.