Modern programming language frameworks, like FastAPI, make it really simple to build high-quality software products quickly. Moreover, using these frameworks, the development process becomes more fun and less tedious.
That said, FastAPI is a new Python web framework that is both strong and delightful. FastAPI is a sophisticated, fast (high-performance) web framework for developing Python 3.6+ APIs with standard Python-type hints.
When combined with React on the front end and MongoDB as the datastore, it creates a complete web application stack named FARM (FastAPI, React, and MongoDB). It is easy to learn and outperforms the MERN stack (MongoDB, Express, React, Node.js) on benchmarks.
Do you want to use FastAPI with MongoDB for your web application? If the answer is “Yes,” you landed on the correct page. In this blog post, you will learn about FastAPI MongoDB integration. Moreover, a brief introduction to FastAPI and MongoDB is also presented.
Table of Contents
What is FastAPI?
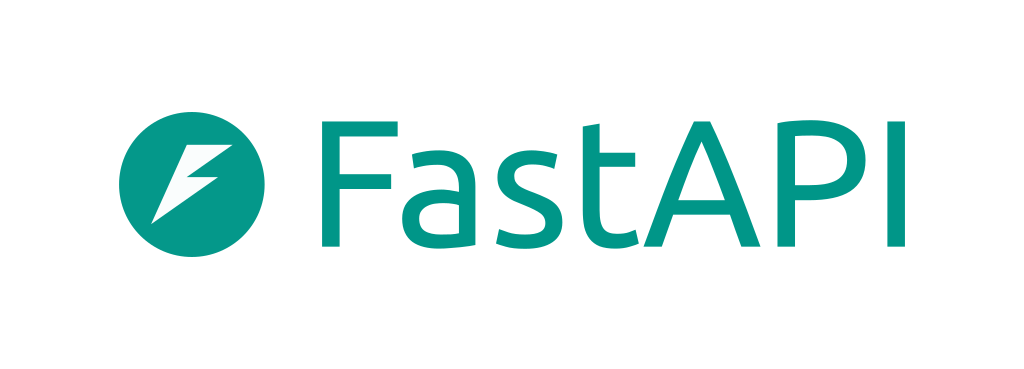
FastAPI is a lighter, fast Python framework for creating APIs. As the name suggests, “FastAPI” is a high-speed and easy-to-develop API that automatically makes well-swagger documentation for your API. FastAPI was created by Sebastián Ramrez and was released in 2018.
FastAPI’s core use case is creating API endpoints, delivering Python dictionary data as JSON, or utilizing the OpenAPI standard, which includes an interactive Swagger UI. FastAPI is still in its early stages but is already used at firms like Uber, Netflix, and Microsoft.
For more information about FastAPI, see the official website.
Hevo Data allows you to seamlessly connect your MongoDB database or REST API sources to any destination of your choice. Whether you’re moving data to a new database, analytics platform, or other formats, our platform ensures a smooth and efficient integration process.
Why Choose Hevo Data?
- Integrate data from 150+ sources(60+ free sources).
- Simplify data mapping and transformations using features like drag-and-drop.
- Easily migrate different data types like CSV, JSON, etc., with the auto-mapping feature.
Join 2000+ happy customers like Whatfix and Thoughtspot, who’ve streamlined their data operations. See why Hevo is the #1 choice for building modern data stacks.
Get Started with Hevo for FreeWhat is MongoDB?
MongoDB is a high-performance document-oriented database with a NoSQL framework. It utilizes collections (tables) with various documents (records) and allows the user to store data in a non-relational fashion.
MongoDB saves its data as objects frequently referred to as documents. These documents are held in collections, similar to how tables function in relational databases. MongoDB is well-known for its scalability, ease of use, dependability, and lack of a requirement to utilize a single schema across all stored documents, allowing them to contain varied fields (columns).
For more information about MongoDB, see the official website.
Why is FastAPI MongoDB Connection Useful?
All web frameworks must bridge the gap between functionality and developer flexibility. FastAPI is closer to Flask in terms of functionality, yet it strikes a better balance. With the FastAPI MongoDB integration, you can leverage all advantages of FastAPI and MongoDB together as a developer.
The following are the crucial advantages of FastAPI MongoDB integration :
- Fast: Application development with FastAPI is extremely fast, comparable to NodeJS and Go. It is known as one of the quickest Python frameworks on the market.
- Fast coding: Speed up the feature development pace by 200 to 300 percent.
- Intuitive: Excellent editing assistance. Debugging takes less time.
- Simple: Designed to be simple to use and understand. Spend less time reading documents.
- Strong: Developer can obtain production-ready code. With interactive documentation generated automatically.
- Standards-based: Based on (and completely compatible with) the open API standards OpenAPI (formerly Swagger) and JSON Schema.
We’ve seen how FastAPI is the most capable web framework, and MongoDB is the most robust and flexible NoSQL database. As a developer, you can leverage the versatility of MongoDB to work with almost any type of data and the unbeatable features of FastAPI to develop fast and secure web applications.
Steps for FastAPI MongoDB Integration
To demonstrate how FastAPI MongoDB integration works, we will build a Cleaning CRUD API with FastAPI and MongoDB.
Prerequisites
- Python 3.9..0 or 3.10+.
- A MongoDB Atlas cluster.
- Basic knowledge of FastAPI
- Knowledge about Pydantic library
- Visual Studio Code Editor
If you have not downloaded and installed MongoDB yet, download it from the official website.
Step 1: Initial Setup and Creating Virtual Environment
Now you are ready to start your project. First, let’s create a new folder to store the project named “fastapi-mongo-demo.” Use the following command to create a new folder.
$ mkdir fastapi-mongo-demo
Let’s open this folder in Visual Studio Code Editor.
Create a virtual environment in your project using the following command.
python -m venv env;
Activate the virtual environment by running the command given below.
env/scripts/activate
Step 2: Install Dependencies
- Install the FastAPI package using the following pip command.
pip install fastapi
- Install the PyMongo package to connect with MongoDB.
pip install pymongo
- As FastAPI uses the Starlette framework for web requests, you must install an ASGI server. Install the ASGI server for FAstAPI using the following command.
pip install uvicorn
Note: Unicorn is just as quick as the server implementation, which aids in the interaction between your application and the backend.
Step 3: Create Root End Point for FastAPI
Now you have successfully installed the required dependencies, let’s develop our first API program for our root endpoint (homepage). First, create the main.py
file in VS Code.
Enter the following code into main.py
.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def index():
return {"message": "Welcome To FastAPI World"}
In the next step let’s run and test our API:
To run the code write the following command in the terminal;
uvicorn main:app --reload
As a result of the above command, your API should be running on localhost at http://127.0.0.1:8000
In your browser, go to http://localhost:8000
. You should get the following output.
{
"message": "Welcome To FastAPI World"
}
Kudos you have successfully built your API 🎉🎉
The interactive API documentation is also available at http://localhost:8000/docs
. You will get the automatically created documentation of your API at the above link. You must get the following similar image as in the output.
Step 4: Configuring MongoDB Atlas
Now you have successfully created your first FastAPI program. In the next step, let’s set up our MongoDB Atlas to store our cleaning service data.
- Sign up for your free MongoDB cloud storage.
- Create a project and name it ‘cleaningstore’ after signing up.
- Click on the ‘Build a Cluster‘ button. After filling required details your clusters will be created within 1-4minutes.
- After creating your cluster, connect it with Cloud storage.
- While choosing the connection method, select “Connect your application.”
- To use your cluster connection, copy the connection string that appears after selecting Python as the driver.
- Save your username and password; it will be used in the next step.
Congratulations, you have successfully prepared and configured our MongoDB Atlas.
Step 5: Create Add User End Point
After creating and configuring cloud storage for your application. In the next step, you will be creating an endpoint. A MongoDB connection URI is required to connect the FastAPI application with the MongoDB cluster. So let’s store the required URI in a file.
Create a settings.py
file and put the following code in it:
# MongoDB attributes
mongodb_uri = 'mongodb+srv://kim:123CloudClean@cluster0.5xmt6.mongodb.net/<usersdata>?retryWrites=true&w=majority'
port = 8000
Ensure you add your correct username and password to the connection string mentioned above.
Now create a database.py
file and write the following code in it. This is a connection file that establishes a connection with MongoClient. Along with the connection, a database name “customerdata” will also be created.
from pymongo import MongoClient
import settings
client = MongoClient(settings.mongodb_uri, settings.port)
db = client[customerdata]
Remember, you created the root endpoint in Step 3. Now you are going to create another endpoint named “addUser.”
from fastapi import FastAPI
import connection
from bson import ObjectId
from schematics.models import Model
class Customer(Model):
cust_id= ObjectId()
cust_email = EmailType(required=True)
cust_name = StringType(required=True)
# An instance of class User
newuser = Customer()
# funtion to create and assign values to the instanse of class Customer created
def create_user(email, username):
newuser.cust_id = ObjectId()
newuser.cust_email = email
newuser.cust_name = username
return dict(newuser)
app = FastAPI()
# Our root endpoint
@app.get("/")
def index():
return {"message": "Welcome to FastAPI World"}
# Signup endpoint with the POST method
@app.post("/signup/{email}/{username}")
def addUser(email, username: str):
user_exists = False
data = create_user(email, username)
# Covert data to dict so it can be easily inserted to MongoDB
dict(data)
# Checks if an email exists from the collection of users
if connection.db.users.find(
{'email': data['email']}
).count() > 0:
user_exists = True
print("Customer Exists")
return {"message":"Customer Exists"}
# If the email doesn't exist, create the user
elif user_exists == False:
connection.db.users.insert_one(data)
return {"message":"User Created","email": data['email'], "name": data['name']}
In the above code, you created the Signup endpoint. This endpoint simply creates a new user in the MongoDB store and returns information about the newly generated user or if the user already exists.
Run your code to check API is working. Type the following command in the terminal.
uvicorn main:app --reload
CRUD Operations in FastAPI with MongoDB
CRUD (Create, Read, Update, Delete) operations are fundamental when working with APIs. Below is a concise guide to implementing CRUD operations in a FastAPI app integrated with MongoDB using the Motor library for asynchronous database interactions.
Create (POST)
To add new data:
- Use
@router.post
to define the endpoint. - Accept data from the request body and validate it using a Pydantic model.
- Insert the data into the MongoDB collection using
insert_one()
. - Return the inserted document’s ID.
Read (GET)
To fetch all records:
- Use
@router.get
to retrieve data. - Fetch all documents using
find()
and convert_id
(BSON) to a string. - Return a list of documents.
Update (PUT)
To modify an existing document:
- Use
@router.put
to define the endpoint. - Accept an
id
parameter and data from the request body. - Use MongoDB’s
update_one()
to update fields in the specified document.
Delete (DELETE)
To remove a document:
- Use
@router.delete
with the document’sid
as a parameter. - Convert the
id
to BSON format and delete the document usingdelete_one()
.
Example Code Snippet
from fastapi import FastAPI, APIRouter, Request, Body
from bson import ObjectId
from pydantic import BaseModel
app = FastAPI()
router = APIRouter()
class Item(BaseModel):
name: str
description: str
@router.post("/")
async def create_item(request: Request, item: Item):
db = request.app.mongodb["collection_name"]
result = await db.insert_one(item.dict())
return {"id": str(result.inserted_id)}
@router.get("/")
async def read_items(request: Request):
db = request.app.mongodb["collection_name"]
items = await db.find({}).to_list(100)
for item in items:
item["_id"] = str(item["_id"])
return items
@router.put("/{id}")
async def update_item(request: Request, id: str, item: Item):
db = request.app.mongodb["collection_name"]
result = await db.update_one({"_id": ObjectId(id)}, {"$set": item.dict()})
return {"updated_count": result.modified_count}
@router.delete("/{id}")
async def delete_item(request: Request, id: str):
db = request.app.mongodb["collection_name"]
result = await db.delete_one({"_id": ObjectId(id)})
return {"deleted_count": result.deleted_count}
Key Considerations
- Data Validation: Use Pydantic models to validate incoming JSON data.
- Error Handling: Handle exceptions like invalid
id
or missing keys. - Swagger UI: Test endpoints at
/docs
or/redoc
in your FastAPI app.
You can also take a look at how you can perform Python REST API Operations to get a better understanding of how REST APIs work.
Conclusion
In this blog, you have learned about FastAPI MongoDB integration. FastAPI is an excellent, fast framework for creating an API. If you are unfamiliar with it, it’s the right time to start learning and exploring it. FastAPI seamlessly connects with MongoDB, simplifying development.
Apart from MongoDB, you would use several applications and databases across your business for Marketing, Accounting, Sales, Customer Relationship Management, etc. It is essential to consolidate data from all these sources to get a complete overview of your business performance. To achieve this, you need to assign a portion of your Engineering Bandwidth to Integrate Data from all sources, Clean & Transform it, and finally, Load it to a Cloud Data Warehouse or a destination of your choice for further Business Analytics. All of these challenges can be comfortably solved by a Cloud-Based ETL tool such as Hevo Data.
Sign up for a 14-day free trial and simplify your data integration process. Check out the pricing details to understand which plan fulfills all your business needs.
Frequently Asked Questions
1. What is the role of MongoDB Atlas in FastAPI MongoDB integration?
MongoDB Atlas provides a cloud-based database service, enabling you to easily connect and manage MongoDB with FastAPI.
2. Can FastAPI handle CRUD operations with MongoDB?
Yes, FastAPI supports CRUD operations (Create, Read, Update, Delete) when integrated with MongoDB using proper endpoints and queries.
3. Why should I use Pydantic in FastAPI MongoDB projects?
Pydantic helps validate and serialize data efficiently, ensuring consistent JSON structure when working with MongoDB and FastAPI.