MongoDB database is a NoSQL, general-purpose program that uses JSON-like documents to store its data. The JSON-like documents provide a flexible and dynamic schema while maintaining simplicity, unlike relational databases that use tabular relationships. Since NoSQL databases can scale horizontally, they are suitable for real-time and big data applications.
Typically, a database driver connects an application with a database program. The communication channel is established by a driver when performing MongoDB CRUD operations in Python. The recommended MongoDB driver for Python is PyMongo.
The aim of this guide is to teach you how to perform the CRUD operations in MongoDB. By the end of this guide, you will understand the fundamentals of how data moves across MongoDB and Python applications.
Table of Contents
What is MongoDB?
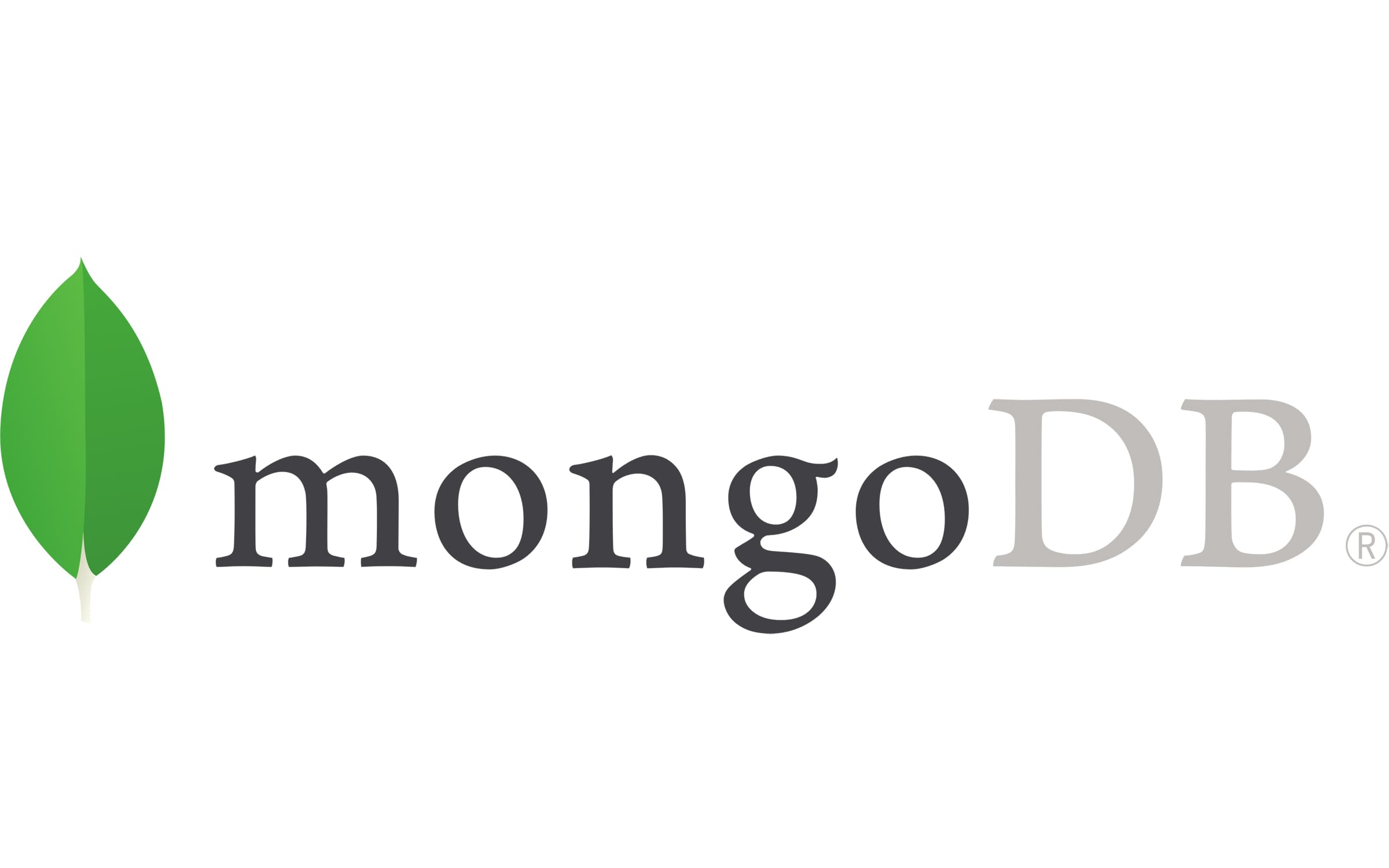
MongoDB is a NoSQL open-source, document-oriented database developed for storing and processing high volumes of data. Compared to conventional relational databases, MongoDB makes use of collections and documents instead of tables consisting of rows and columns. The collections consist of several documents containing the basic units of data in terms of key and value pairs.
Introduced in February 2009, the MongoDB database is designed, maintained, and managed by MongoDB.Inc under SSPL(Server Side Public License). Organizations such as Facebook, Nokia, eBay, Adobe, Google, etc. prefer it for efficiently handling and storing their exponentially growing data.
MongoDB offers official driver support for programming languages such as C, C++, C#, Go, Java, Node.js, Perl, PHP, Python, Motor, Ruby, Scala, Swift, and Mongoid. If you’re looking for a viable database where you can store data in flexible, JSON-like documents, you can’t go wrong with MongoDB.
In this guide, we’ll be covering MongoDB CRUD operations in Python using the Python driver- PyMongo. If you would like to learn about CRUD Operations in MongoDB using C#, we have a separate blog piece here- Get Started with CRUD Operations in MongoDB using C#: 3 Easy Steps.
For more information on MongoDB features and tools, have a look at our comprehensive guide- Best MongoDB Tools in 2022: Tools & Data Engineer Must Know.
What Does CRUD Mean in MongoDB?
A CRUD operation in MongoDB describes a user interface that allows users to view, search, and modify data in a database.
Modifications to MongoDB documents are performed by connecting to a server, querying the correct documents, and changing the settings before sending them back to the database. CRUD is a data-oriented, HTTP action verb-based process.
CRUD literally stands for Create, Read, Update, and Delete.
- Create inserts new documents into the MongoDB database.
- Read operation is used to retrieve documents from a database.
- Update operation modifies existing documents in the database.
- Delete operation removes documents from a database.
Prerequisites for Setting Up MongoDB CRUD Operations in Python
In this MongoDB CRUD operations in Python guide, you’ll need the following:
- The latest version of Python, i.e. Python 3.6+.
- MongoDB database.
- PyMongo MongoDB driver installation.
If you are a new user, you can explore our easy-to-follow instructions on installing MongoDB GUI for your platform.
How to Perform MongoDB CRUD Operations in Python?
We have now defined MongoDB’s CRUD operations, so let’s see how to perform each MongoDB CRUD Operation in Python, and manipulate documents in MongoDB. Before moving directly to the CRUD Operations, let’s have a look at how you can set up MongoDB CRUD operations in Python.
Setting Up MongoDB CRUD Operations in Python
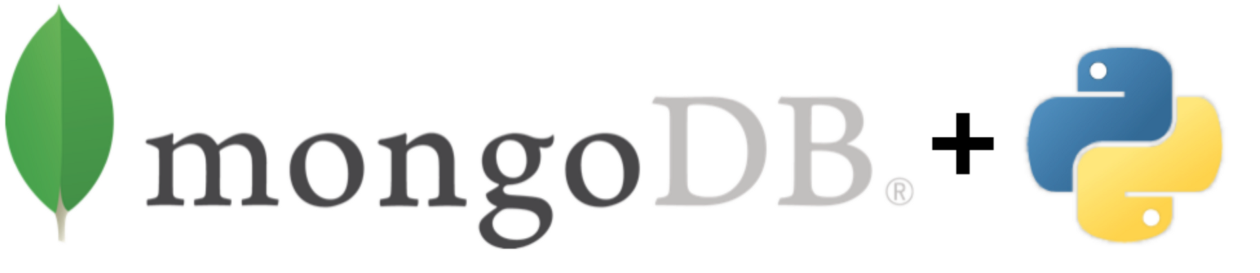
MongoDB provides its users a python distribution called PyMongo that contains tools for working with MongoDB databases. To install PyMongo for communicating with MongoDB, follow these steps:
In your command prompt, type the following command to install PyMongo.
pip install pymongo
Now to perform MongoDB CRUD operations in Python, you need to establish a connection using Mongo client. Type in the following command:
>>> from pymongo import MongoClient
>>> client = MongoClient('localhost',27017) # 27017 is the default port number for mongodb
This MongoClient method is used to connect to the MongoDB server. Next, connect to your test database:
db = client.test
Retrieve the collection from the database:
col = db.person
You’re all set to perform MongoDB CRUD operations in Python. Let’s take a look at the process of creating, reading, updating, and deleting documents, one by one.
Using MongoDB CRUD Operations in Python
C-Create Operation
Data is stored in MongoDB as JSON objects. The records for a collection in MongoDB are referred to as documents. The Create operation in MongoDB creates a new collection and it is executed if a given collection does not exist. MongoDB creates only one collection at a time, not multiple collections. The insert operation in MongoDB is atomic for each document.
There are two ways you can add documents to a MongoDB collection.
- db.collection.insert_one()
- db.collection.insert_many()
insert_one()
insert_one() facilitates the insertion of a single document into a collection. We’ll be working with a collection called SalesDB in this example.
By calling the insert_one() method on SalesDB, we can add a single entry to our collection. We then establish a schema by providing the key-value pair information we want to insert.
db.SalesDB.insert_one({
name: "Linda",
orderdate: "6/10/2021",
species: "Dog",
ownerAddress: "380 W. Fir Ave",
chipped: true
})
Upon successful completion of the create operation, a new document is created. The function will return an object whose “acknowledged” attribute is “true” and whose “insertedID” attribute is the newly created “ObjectId.”
> db.SalesDB.insert_one({
... name: "Linda",
… orderdate: "6/10/2021",
… species: "Dog",
… ownerAddress: "380 W. Fir Ave",
… chipped: true
... })
{
"acknowledged" : true,
"insertedId" : ObjectId("5fd989674e6b9ceb8665c57d")
}
insert_many()
Using the insert_many() method on a collection, it’s possible to insert multiple documents at the same time. Here, we separate multiple items using commas and pass them into our chosen collection- SalesDB.
Using brackets between parentheses indicates that multiple entries are being passed in. Such a method is often called a nested method.
db.SalesDB.insert_many([{
name: "Linda",
orderdate: "6/10/2021",
species: "Dog",
ownerAddress: "380 W. Fir Ave",
chipped: true},
{name: "Kitana",
orderdate: "6/10/2021",
species: "Cat",
ownerAddress: "521 E. Cortland",
chipped: true}])
db.SalesDB.insert_many([{ name: "Linda", orderdate: "6/11/2021", species: "Dog",
ownerAddress: "380 W. Fir Ave", chipped: true}, {name: "Kitana", orderdate: "6/11/2021",
species: "Cat", ownerAddress: "521 E. Cortland", chipped: true}])
{
"acknowledged" : true,
"insertedIds" : [
ObjectId("5fd98ea9ce6e8850d88270b4"),
ObjectId("5fd98ea9ce6e8850d88270b5")
]
}
R-Read Operation
When you read documents, you can specify which documents you want based on special filters and criteria. MongoDB’s documentation provides more information about these query filters. Additionally, query modifiers can be used to alter the number of results returned.
There are two ways to read documents from a collection in MongoDB:
- db.collection.find()
- db.collection.find_one()
find()
We can use find() method, one of the MongoDB CRUD operations in Python, on a collection to get all the documents within it. Using the find() method without any arguments will return all records in the collection.
db.SalesDB.find()
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
{ "_id" : ObjectId("5fd993a2ce6e8850d88270b7"), "name" : "Marsh", "orderdate" : "6/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
{ "_id" : ObjectId("5fd993f3ce6e8850d88270b8"), "name" : "Loo", "orderdate" : "7/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
{ "_id" : ObjectId("5fd994efce6e8850d88270ba"), "name" : "Kevin", "orderdate" : "8/10/2021", "species" : "Dog", "ownerAddress" : "900 W. Wood Way", "chipped" : true }
The “ObjectId” assigned to every record is mapped to the “_id” key.
When performing a read operation, you can use filtering criteria to narrow your search to a desired subsection of the records. Searching by value is one of the most common ways to filter the results.
db.SalesDB.find({"species":"Cat"})
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
find_one()
If we only want one document that meets the search criteria, then we can use the find_one() method on the collection. Whenever more than one document matches the query, this method returns the document that reflects the order of the documents on disk, according to their natural order.
A null value is returned if none of the documents match the query. The syntax of the function is as follows.
db.{collection}.find_one({query}, {projection})
Consider the following collection as an example.
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
{ "_id" : ObjectId("5fd993a2ce6e8850d88270b7"), "name" : "Marsh", "orderdate" : "6/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
{ "_id" : ObjectId("5fd993f3ce6e8850d88270b8"), "name" : "Loo", "orderdate" : "7/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
{ "_id" : ObjectId("5fd994efce6e8850d88270ba"), "name" : "Kevin", "orderdate" : "8/10/2021", "species" : "Dog", "ownerAddress" : "900 W. Wood Way", "chipped" : true }
Here is the line of code we run:
db.SalesDB.find({"orderdate":"8/10/2021"})
In this case, we would get the following result:
{ "_id" : ObjectId("5fd994efce6e8850d88270ba"), "name" : "Kevin", "orderdate" : "8/10/2021", "species" : "Dog", "ownerAddress" : "900 W. Wood Way", "chipped" : true }
U-Update Operation
Update operations happen at the level of a single document, just like create MongoDB CRUD operations in Python, update operations operate on a single collection. Filters and criteria are used to select the documents to be updated in an update operation.
Document updates are permanent and cannot be rolled back. Be careful when updating documents. This also applies to deleting documents.
MongoDB CRUD allows users to update documents in three different ways:
- db.collection.update_one()
- db.collection.update_many()
- db.collection.replace_one()
update_one()
By performing an update_one() operation, we can change a single document and update a record. This is done using the update_one() method on a collection, which here is “SalesDB.” To update a document, two arguments need to be provided: an update filter and an update action.
Update filters specify which items should be updated, and update actions specify how to do so. We start by passing the update filter. Our next step is to use the “$set” key and provide the value of the fields we wish to update. If the provided filter matches the existing record, the update will be applied.
db.SalesDB.update_one({name: "Marsh"}, {$set:{ownerAddress: "451 W. Coffee St. A204"}})
{ "acknowledged" : true, "matchedCount" : 1, "modifiedCount" : 1 }
{ "_id" : ObjectId("5fd993a2ce6e8850d88270b7"), "name" : "Marsh", "orderdate" : "6/10/2021", "species" : "Dog", "ownerAddress" : "451 W. Coffee St. A204", "chipped" : true }
update_many()
The update_many() function allows us to update multiple documents by passing in a list of items, just as we did when we inserted multiple items. In this case, we use the same update syntax as when we updated a single document.
db.SalesDB.update_many({species:"Dog"}, {$set: {age: "5"}})
{ "acknowledged" : true, "matchedCount" : 3, "modifiedCount" : 3 }
> db.SalesDB.find()
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
{ "_id" : ObjectId("5fd993a2ce6e8850d88270b7"), "name" : "Marsh", "orderdate" : "6/10/2021", "species" : "Dog", "ownerAddress" : "451 W. Coffee St. A204", "chipped" : true }
{ "_id" : ObjectId("5fd993f3ce6e8850d88270b8"), "name" : "Loo", "orderdate" : "7/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
{ "_id" : ObjectId("5fd994efce6e8850d88270ba"), "name" : "Kevin", "orderdate" : "8/10/2021", "species" : "Dog", "ownerAddress" : "900 W. Wood Way", "chipped" : true }
replace_one()
The replace_one() method replaces a single document in a collection. The replace_one() method replaces a document’s entire contents, so fields not found in the new document will be lost.
db.SalesDB.replace_one({name: "Kevin"}, {name: "Maki"})
{ "acknowledged" : true, "matchedCount" : 1, "modifiedCount" : 1 }
> db.SalesDB.find()
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
{ "_id" : ObjectId("5fd993a2ce6e8850d88270b7"), "name" : "Marsh", "orderdate" : "6/10/2021", "species" : "Dog", "ownerAddress" : "451 W. Coffee St. A204", "chipped" : true }
{ "_id" : ObjectId("5fd993f3ce6e8850d88270b8"), "name" : "Loo", "orderdate" : "7/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
{ "_id" : ObjectId("5fd994efce6e8850d88270ba"), "name" : "Maki" }
D-Delete Operation
The delete operation operates on a single collection, like the update and create operations. Deletes are also atomic for a single document. Delete operations can be specified with filters and criteria in order to specify which documents you want to remove from a collection. The criteria and filters work in the same way as filter options in read MongoDB CRUD operations in Python.
There are two ways to delete records from a collection in MongoDB:
- db.collection.delete_one()
- db.collection.delete_many()
delete_one()
The delete_one() method is used to remove a document from a MongoDB collection. The document to be deleted is specified by a filter. Only records matching the specified filter are deleted.
db.SalesDB.delete_one({name:"Maki"})
{ "acknowledged" : true, "deletedCount" : 1 }
> db.SalesDB.find()
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
{ "_id" : ObjectId("5fd993a2ce6e8850d88270b7"), "name" : "Marsh", "orderdate" : "6/10/2021", "species" : "Dog", "ownerAddress" : "451 W. Coffee St. A204", "chipped" : true }
{ "_id" : ObjectId("5fd993f3ce6e8850d88270b8"), "name" : "Loo", "orderdate" : "7/10/2021", "species" : "Dog", "ownerAddress" : "380 W. Fir Ave", "chipped" : true }
delete_many()
A single delete operation is used to delete multiple documents from a desired collection with delete_many(). The method takes a list as a parameter and filters each item according to filter criteria as in delete_one().
db.SalesDB.delete_many({species:"Dog"})
{ "acknowledged" : true, "deletedCount" : 2 }
> db.SalesDB.find()
{ "_id" : ObjectId("5fd98ea9ce6e8850d88270b5"), "name" : "Kitana", "orderdate" : "6/10/2021", "species" : "Cat", "ownerAddress" : "521 E. Cortland", "chipped" : true }
Learn More About:
NoSQL CRUD Operations Made Easy
Conclusion
In this guide, we have explored how to perform MongoDB CRUD operations in Python, which is creating, retrieving, updating, and deleting documents. Despite the focus of this guide on introductory concepts, MongoDB offers more powerful and flexible tools for working with non-relational databases. You can also connect additional analytical applications to run more comprehensive operations on your data and analyze it in greater detail!
If you use MongoDB database on a recurrent basis and wish to transfer your database files to a secure single location, a Cloud-based Data Warehouse might be the best alternative.
Worried about where to start with? A simple and speedy data transfer solution like Hevo ETL helps you in that.
Hevo Data with its strong integration with 100+ Sources & BI tools such as MongoDB allows you to not only export data from sources & load data in the destinations, but also transform & enrich your data, & make it analysis-ready so that you can focus only on your key business needs and perform insightful analysis using BI tools.
Visit our Website to Explore HevoHevo lets you migrate your data from your MongoDB database to any Data Warehouse of your choice like Amazon Redshift, Snowflake, Google BigQuery, or Firebolt, within minutes with just a few clicks.
Why not give Hevo a try? Sign Up here for a 14-day free trial and experience the feature-rich Hevo suite first hand. You can also check out our unbeatable pricing and make a decision on your best-suited plan.
Share your ideas about learning MongoDB CRUD Operations in Python in the comments area below. Tell us of any other MongoDB-supported programming languages you’d want us to cover. We’d love to hear your thoughts and ideas.
Frequently Asked Questions
1. What are MongoDB CRUD operations?
CRUD operations are the four basic operations that you can perform on a database: Create, Read, Update, and Delete.
2. What is CRUD operations in Python?
In Python, CRUD operations involve interacting with a database to perform the following: Create, Read, Update, Delete
3. How to query data from MongoDB using Python?
To perform CRUD operations on a MongoDB database using Python, you typically use the pymongo library, which provides a set of tools to work with MongoDB.