Facebook is the most popular social networking site on the Internet. It was co-created by Mark Zuckerberg and officially launched on February 4, 2004. Facebook is a popular destination for users to set up their personal web pages, connect with friends, share pictures, share movies, talk about what they’re doing, etc.
Table of Contents
Introduction to Webhooks
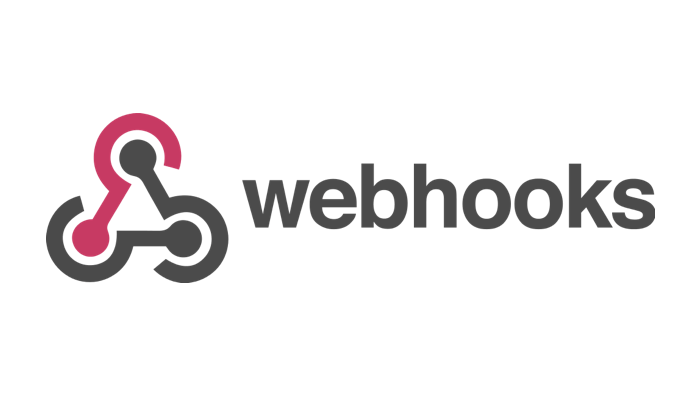
Webhooks are automated messages sent from apps to your devices when something gets triggered inside the application interface. They have a message—or payload—and are sent to a unique URL—essentially the app’s phone number or address. Webhooks are almost always faster than polling and require less work on your end. They’re much like SMS notifications.
A webhook is a software architecture approach that allows applications and services to submit a web-based notification to other applications whenever a specific event occurs. The application provides a way for users to register or connect application programming interface calls to certain events under specific conditions, such as when a new user, account, or order is created or an order ships out of a warehouse.
Benefits of webhooks
Because webhooks notify other systems when an event occurs, changes are event-driven and made in near-real-time. A continuous integration system using webhooks might send a message to the security team only when a build failure is due to a security problem, for example. This can “push” information onto the screens of the security team, instead of causing them to search for it periodically in other systems. It can also allow systems to synchronize in near-real-time, instead of overnight or in a batch.
An alternative to a webhook is a polling process. A polling process has a program wait five or 10 minutes, then calls an API for a list of recent transactions, adding any new ones to a list and processing them. This assumes the API provides a list of recent transactions.
Uses and examples of webhooks
Webhooks are a simple way to make an API accessible to make or receive calls or send text-based responses to users when specific events occur within the application. Platforms that support webhooks include Facebook, Github, Trello, Confluence, and Google Calendar.
Practical uses of webhooks can include:
- Daily automatic email reminders for meetings
- Confirmations of processed and completed payments
- Sync changes in customer data between applications
Webhooks are very common on the internet of things (IoT), where a sensor might notice a change in temperature and call the air conditioning system. A motion sensor could send a message to a security system or generate noise to simulate a barking dog, for example.
Introduction to Facebook App
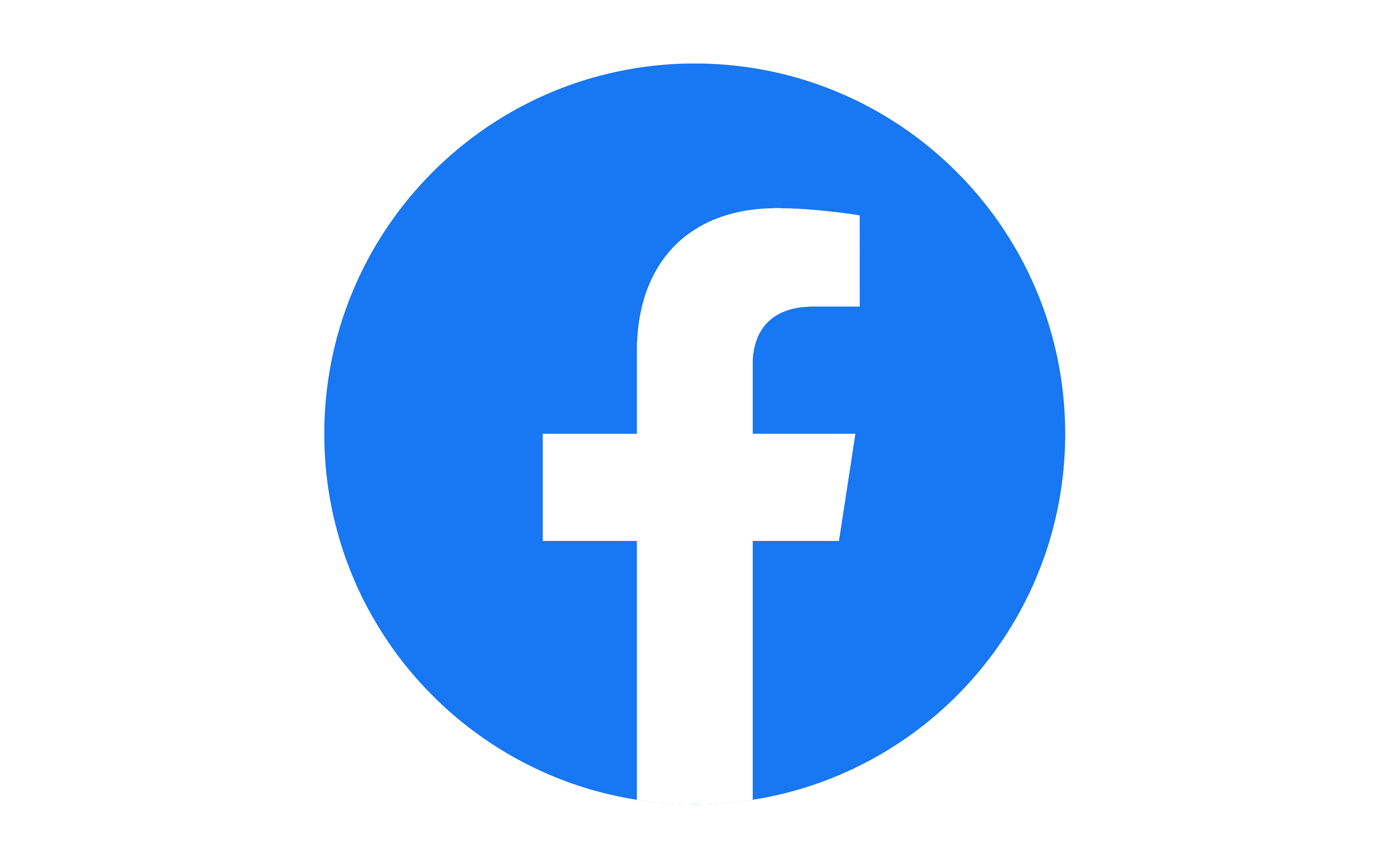
Facebook is a social networking website where users can post comments, share photographs, and post links to news or other interesting content on the web, chat live, and watch short-form videos. Shared content can be made publicly accessible, or it can be shared only among a select group of friends or family, or with a single person.
Facebook makes it easy for you to connect with family and friends online. Originally designed for college students, Facebook was created in 2004 by Mark Zuckerberg while he was enrolled at Harvard University. By 2006, anyone over the age of 13 with a valid email address could join Facebook. Today, Facebook is the world’s largest social network, with more than 1 billion users worldwide.
Key Features of Facebook
Here are a few features that make Facebook so popular:
- Facebook allows you to maintain a friends list and choose privacy settings to tailor who can see content on your profile.
- Facebook allows you to upload photos and maintain photo albums that can be shared with your friends.
- Facebook supports interactive online chat and the ability to comment on your friend’s profile pages to keep in touch, share information, or say “hi.”
- Facebook supports group pages, fan pages, and business pages that let businesses use Facebook as a vehicle for social media marketing.
- Facebook’s developer network delivers advanced functionality and monetization options.
- You can stream videos live using Facebook Live.
- Chat with Facebook friends and family members, or auto-display Facebook pictures with the Facebook Portal device.
How to set up Facebook Webhooks Integration?
Facebook Webhooks Integration is a two-part process.
- Facebook Webhooks Integration: Setting up Webhooks
- Facebook Webhooks Integration: Setting up Facebook App
Facebook Webhooks Integration: Setting Up Your Webhook
Your Facebook webhook is the core of your Messenger experience. This is where your code lives, and where you will receive, process, and send messages.
In this guide, you will learn how to set up a basic Facebook webhook that supports the Messenger Platform’s required Facebook webhook verification step and is able to accept Facebook webhook events.
Requirements
To follow this guide to set up your Facebook webhook, all you need is a computer with Node.js installed.
To deploy a live Facebook webhook that can receive Facebook webhook events from the Messenger Platform, your code must be hosted on a public HTTPS server that meets the following requirements:
- HTTPS support, Self-signed certificates are not supported
- A valid SSL certificate
- An open port that accepts GET and POST requests
Create a new Node.js project
Run the following on the command line to create the needed files and dependencies:
mkdir messenger-webhook // Creates a project directory
cd messenger-webhook // Navigates to the new directory
touch index.js // Creates empty index.js file.
npm init // Creates package.json. Accept default for all questions.
npm install express body-parser --save
// Installs the express.js http server framework module, and then adds them to the dependencies section of package.json file.
If everything went well, your messenger-webhook
the directory should look like this:
index.js
node_modules
package.json
Create an HTTP server
Add the following code to index.js
:
'use strict';
// Imports dependencies and set up http server
const
express = require('express'),
bodyParser = require('body-parser'),
app = express().use(bodyParser.json()); // creates express http server
// Sets server port and logs message on success
app.listen(process.env.PORT || 1337, () => console.log('webhook is listening'));
This code creates an HTTP server that listens for requests on the default port, or port 1337 if there is no default. For this guide, we are using Express, a popular, lightweight HTTP framework, but you can use any framework you love to build your webhook.
Add your webhook endpoint
Add the following code to index.js
:
// Creates the endpoint for our webhook
app.post('/webhook', (req, res) => {
let body = req.body;
// Checks this is an event from a page subscription
if (body.object === 'page') {
// Iterates over each entry - there may be multiple if batched
body.entry.forEach(function(entry) {
// Gets the message. entry.messaging is an array, but
// will only ever contain one message, so we get index 0
let webhook_event = entry.messaging[0];
console.log(webhook_event);
});
// Returns a '200 OK' response to all requests
res.status(200).send('EVENT_RECEIVED');
} else {
// Returns a '404 Not Found' if event is not from a page subscription
res.sendStatus(404);
}
});
This code creates a /webhook
endpoint that accepts POST
requests check a request is a webhook event, then parses the message. This endpoint is where the Messenger Platform will send all webhook events.
Note that the endpoint returns a 200OK
response, which tells the Messenger Platform the event has been received and does not need to be resent. Normally, you will not send this response until you have completed processing the event.
Add webhook verification
Add the following code to index.js
:
// Adds support for GET requests to our webhook
app.get('/webhook', (req, res) => {
// Your verify token. Should be a random string.
let VERIFY_TOKEN = "<YOUR_VERIFY_TOKEN>"
// Parse the query params
let mode = req.query['hub.mode'];
let token = req.query['hub.verify_token'];
let challenge = req.query['hub.challenge'];
// Checks if a token and mode is in the query string of the request
if (mode && token) {
// Checks the mode and token sent is correct
if (mode === 'subscribe' && token === VERIFY_TOKEN) {
// Responds with the challenge token from the request
console.log('WEBHOOK_VERIFIED');
res.status(200).send(challenge);
} else {
// Responds with '403 Forbidden' if verify tokens do not match
res.sendStatus(403);
}
}
});
This code adds support for the Messenger Platform’s webhook verification to your Facebook webhook. This is required to ensure your Facebook webhook is authentic and working.
The verification process looks like this:
- You create a verify token. This is a random string of your choosing, hardcoded into your webhook.
- You provide your verify token to the Messenger Platform when you subscribe to your webhook to receive webhook events for an app.
- The Messenger Platform sends a
GET
request to your webhook with the token in thehub.verify
parameter of the query string. - You verify the token sent matches your verified token, and respond with
hub.challenge
parameter from the request. - The Messenger Platform subscribes your webhook to the app.
Test your webhook
Now that you have all the code in place for a basic webhook, it is time to test it by sending a couple of sample requests to your Facebook webhook running on localhost.
Run the following on the command line to start your Facebook webhook on localhost:
node index.js
From a separate command line prompt, test your webhook verification by substituting your verify token into this cURL request:
curl -X GET "localhost:1337/webhook?hub.verify_token=<YOUR_VERIFY_TOKEN>&hub.challenge=CHALLENGE_ACCEPTED&hub.mode=subscribe"
If your Facebook webhook verification is working as expected, you should see the following:
WEBHOOK_VERIFIED
logged to the command line where your node process is running.CHALLENGE_ACCEPTED
logged to the command line where you sent the cURL request.
Test your webhook by sending this cURL request:
curl -H "Content-Type: application/json" -X POST "localhost:1337/webhook" -d '{"object": "page", "entry": [{"messaging": [{"message": "TEST_MESSAGE"}]}]}'
If your webhook is working as expected, you should see the following:
TEST_MESSAGE
logged to the command line where your node process is running.EVENT RECEIVED
logged to the command line where you sent the cURL request.
Deploy your webhook
Your Facebook webhook should now be tested and working as expected, but it does you no good running on localhost! The next step is to deploy your webhook code to the server of your choice. This can be a cloud-based service like a Heroku or AWS EC2 instance, or your own hardware.
Once your Facebook webhook is deployed, try running the test cURL requests above again to make sure everything is working as expected. Do not forget to substitute the public URL or IP address of your server for localhost in the sample requests.
Facebook Webhooks Integration: Setting Up Your Facebook App
When your Facebook app is in Development Mode, plugin and API functionality will only work for admins, developers, and testers of the app. After your app is approved and public, it will work for the general public.
A Facebook app is what links your Facebook Page to your Facebook webhook, and is where you will configure various settings for your Messenger experience, generate access tokens, and choose what events are sent to your webhook.
In this guide, you will learn how to set up your Facebook app so that you can start building on the Messenger Platform.
Requirements
Before you begin, you will need to create a few things. Please ensure you have all of the following:
- Facebook Page: A Facebook Page will be used as the identity of your Messenger experience. When people chat with your app, they will see the Page name and the Page profile picture. To create a new Page, visit https://www.facebook.com/pages/create
- Facebook Developer Account: Your developer account is required to create new apps, which are the core of any Facebook integration. You can create a new developer account by going to the Facebook Developers website and clicking the ‘Get Started’ button.
- Facebook App: The Facebook app contains the settings for your Messenger experience, including access tokens. To create a new app, visit your app dashboard.
- Webhook URL: Actions that take place in conversations, such as new messages are sent as events to your webhook. For more information on setting up your webhook, see Set Up Your Webhook.
Add the Messenger Platform to your Facebook app
- In the sidebar of your app settings under ‘PRODUCTS’, click ‘+ Add Product’.
- Hover over ‘Messenger’ to display options.
- Click the ‘Set Up’ button.
The Messenger Platform will be added to your app, and the Messenger settings console will be displayed.
Configure the webhook for your app
- In the ‘Webhooks’ section of the Messenger settings console, click the ‘Setup Webhooks’ button.
- In the ‘Callback URL’ field, enter the public URL for your Facebook webhook.
- In the ‘Verify Token’ field, enter the verify token for your Facebook webhook. See Step 4 of Webhook Setup for further reference into what a verify token is
- Click ‘Verify and Save’ to confirm you callback URL
The Messenger Platform will send a GET
request to your Facebook webhook with the verify token you provided. If your webhook is valid and properly set up to respond to the verification request, your webhook settings will be saved.
Subscribe your app to a Facebook Page
- Click ‘Add or Remove Pages’ and select the pages you want to subscribe your app to. Your webhook will receive events for each selected page when people chat with it through Messenger.
- In the ‘Access Tokens’ section, there will be a Generate Token button for each authorized Page. Make sure to save the access token, it’s needed to send messages using the Send API.
- For each authorized Page, the ‘Webhooks’ section will contain the fields the app can subscribe to. Click on “Add Subscriptions” to select desired fields. At a minimum, we recommend you choose
messages
andmessaging_postbacks
to get started.
The Messenger Platform will now be able to send the Facebook webhook events you subscribed to for the selected Page to your webhook.
Test your app subscription
To test that your app setup was successful, go to Messenger and send a message to your Page. If your callback URL receives a Facebook webhook event, you have fully set up your app for receiving messages!
Conclusion
This article gives a comprehensive guide on Facebook and Webhooks. It also gives a step-by-step guide on setting up Facebook Webhooks Integration.
While Facebook Webhooks Integration is insightful, it is a hectic task to Set Up the proper environment. To make things easier, Hevo comes into the picture. Hevo Data is a No-code Data Pipeline and has awesome 100+ pre-built Integrations that you can choose from.
Share your experience of learning about Integration in the comments section below.