The two major stacks revolving around Javascript are MEAN (MongoDB, Express, Angular & Node) and MERN (MongoDB, Express, React & Node). Both the stacks are well suited for dynamic, extensive applications such as LinkedIn, Netflix, PayPal, etc.
This article will help you connect NodeJS with MongoDB with ease. But beforehand, let’s take a look at both of them in brief.
Table of Contents
What is NodeJS?
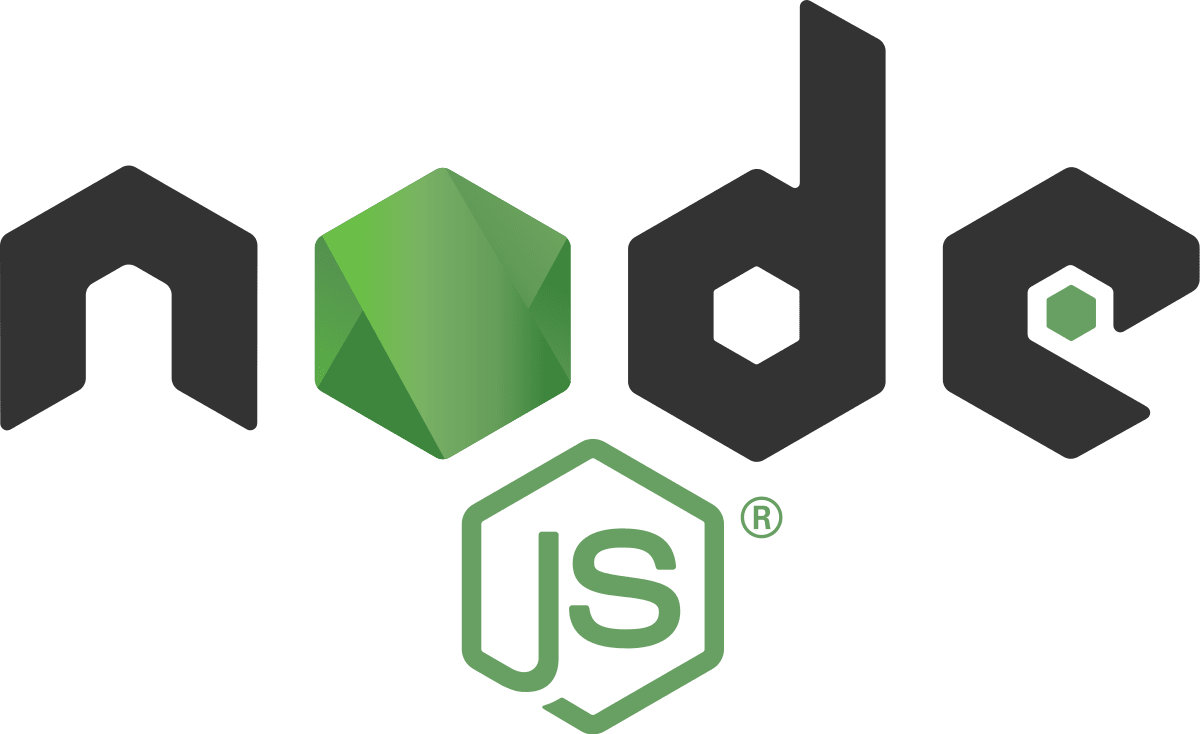
NodeJS is a Javascript runtime built on Chrome’s V8 Javascript engine. NodeJS uses a lightweight, non-blocking event-driven IO model which makes it efficient for asynchronous programming. NodeJS Open Source is a serverless environment that runs JavasScript-based scripts to build scalable applications.
Node.js can be considered as a “JavaScript everywhere” regime i.e., integrate Application Development (web) around a sole Programming Language, rather than different programming media being used for the associated stakeholders i.e., Server-side and Client-side codes. NodeJS is commonly used for event-driven servers, websites, and active back-end API services.
What are MongoDB and Ubuntu?
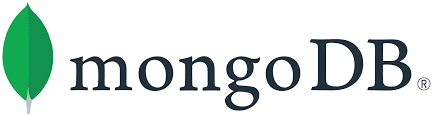
MongoDB is an open-source, document-based database management system. Data in MongoDB is stored in JSON like documents that are schema-less. Thus MongoDB works great in scenarios where you can’t define a fixed data model. It makes use of collections (tables) each having multiple documents (records) & allows the user to store data in a non-relational format. MongoDB is known for its scalability, ease of use, reliability & no compulsion for using a fixed schema among all stored documents, giving them the ability to have varying fields (columns).
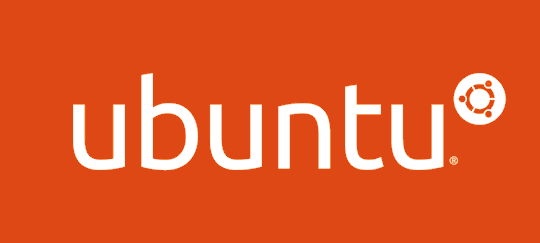
Ubuntu is a robust Linux-based Operating System (OS), available as open-source software. Ubuntu is available free of cost for all users. In addition to that, Ubuntu has a world-class community of Software Developers that ensure that the Operating System remains up-to-date and provides a smooth experience to all its users. Ubuntu can be accessed across a diverse set of platforms and is compatible with all platforms including Computers, Smartphones, and even Servers.
Great! Let’s now look at how to connect NodeJS with MongoDB on Ubuntu 16.04.
Exploring the best databases for 2025? Hevo makes it simple to migrate data from any of these top databases to your desired destinations. Our no-code platform automates data pipelines, ensuring smooth and efficient data transfer.
Why Use Hevo?
- Broad Database Support: Seamlessly connect with a wide range of databases and load data to your preferred destinations.
- Automated Data Migration: Reduce manual effort with automated data syncing and transformation.
- Real-Time Data Flow: Keep your data up-to-date and ready for analysis.
Don’t just take our word for it – see why we’re rated 4.3/5 on G2.
Get Started with Hevo for FreeWhat is NPM?
NPM stands for Node Package Manager and is the default Package Manager for the Node JS platform. NPM is committed to making JavaScript development elegant, productive, and safe. This simplifies the process of finding nodes and managing them easier. It consists of a Command-line Client, also called npm, and an Online Database of public and paid-for private packages, called the npm Registry.
Initial Setup
Following components will be used for connecting NodeJS with MongoDB.
- Ubuntu 16.04 x64
- MongoDB v3.2.16
- NodeJS v6.11.2 LTS
- The MongoDB NodeJS driver
*MongoDB is supported for all stable versions of NodeJS. For the purpose of this blog, we shall be using the above configuration.
How to Install NodeJS for Ubuntu?
To install Nodejs on Ubuntu, add the PPA to your system as shown below.
curl -sL https://deb.nodesource.com/setup_14.x | sudo -E bash -
This ensures that you have successfully added NodeJS PPA in your Ubuntu Operating System.
To install NodeJS on Ubuntu using apt-get, execute the following command. This will also install NPM with NodeJS.
sudo apt-get install -y nodejs
In addition to NPM, this command also installs many other dependent packages on your Ubuntu System.
After installation, you can verify that NodeJS is installed on your system by typing the following command.
node -v
You should see the installed node version as an output.
V6.11.2
For a complete guide to NodeJS installation, you can visit here.
Verify that MongoDB process is running.
ps -ef | grep mongo
You should see an output like
mongodb 25400 1 0 10:57 ? 00:00:01 /usr/bin/mongod --quiet --config /etc/mongod.conf
If MongoDB is not running, execute the following command
sudo service mongod start
Alternatively, you can run the following command from MongoDB bin directory.
mongod
MongoDB comes with an interactive javascript shell. You can use the mongo shell to query and update data as well as perform administrative operations.
Start the shell process by running the following command.
mongo
You will see an output similar to this.
MongoDB shell version: 3.2.16
connecting to: test
Let’s check the list of existing databases by typing.
show dbs
This outputs list of existing dbs along with space occupied
local 0.078GB
security 0.078GB
In this post, we shall be using security. Issue the following command to use security.
use security
Let’s see what’s inside this database.
show collections
Output
system.indexes
Great, everything seems to be working fine, let’s move ahead with integrating NodeJS with MongoDB.
How to Connect NodeJS with MongoDB?
In order to connect NodeJS with MongoDB, we need a MongoDB driver for NodeJS. Install the official MongoDB driver from npm using the following command inside your application directory.
npm install -P mongodb
After a successful installation, we are ready to connect our NodeJS application with MongoDB.
Let’s create a file call app.js that will contain the connection logic. In a file db_connect.js add the following code.
var MongoClient = require('mongodb').MongoClient;
var database = undefined;
var dbUrl = 'mongodb://127.0.0.1:27017/security';
MongoClient.connect(dbUrl, function(err, db) {
if (err) {
throw err;
} else {
database = db;
console.log('MongoDB connection successful');
}
});
MongoClient object exposes a method connect, that returns a database object in callback. It has the following syntax.
connect(url, options, callback)
The first argument is a URL string with the following format.
mongodb://[username:password@]host1[:port1][,host2[:port2],...[,hostN[:portN]]][/[database][?options]]
The second argument is an object literal that specifies optional settings like autoReconnect, poolSize, etc.
The third argument is a callback function that provides errors on connection failure or database objects in case of a successful response.
Try executing this file by entering the following command from your application directory.
node db_connect.js
If the connection is successful, you will see console output as:
MongoDB Connection Successful
Cool. So, we have successfully connected to the security database. Let’s try to insert some data in a new collection called users. Modify db_connect.js as below.
var MongoClient = require('mongodb').MongoClient;
var database = undefined;
var dbUrl = 'mongodb://127.0.0.1:27017/security';
MongoClient.connect(dbUrl, function(err, db) {
if (err) {
throw err;
} else {
database = db;
console.log('MongoDB connection successful');
var testUser = {
name: 'Suraj Poddar'
};
var users = db.collection('users');
users.insert(testUser, function(err, docs) {
if (err) {
throw err;
} else {
console.log(docs);
}
});
}
});
Upon successfully connecting NodeJS with MongoDB, the output will look something like this.
MongoDB connection successful
{ result: { ok: 1, n: 1 },
ops: [ { name: 'Suraj Poddar', _id: 59ad4719eb794f64c84e7ba6 } ],
insertedCount: 1,
insertedIds: [ 59ad4719eb794f64c84e7ba6 ] }
Awesome! We were successfully able to connect to a MongoDB server, read and write to it. For a complete list of APIs provided by MongoDB driver for NodeJS look for the `Releases` section here.
Conclusion
This article teaches you how to connect NodeJS with MongoDB with ease and answers all your queries regarding it. It provides a brief introduction of various concepts related to it & helps the users understand them better. These methods, however, can be challenging especially for a beginner & this is where Hevo saves the day.
Hevo Data, a No-code Data Pipeline can help you replicate data from various 150+ data sources to your desired destination in real-time, without having to write any code. It also helps you transform & enrich your data, & make it analysis-ready so that you can focus only on your key business needs and perform insightful analysis using BI tools. Hevo being a fully-managed system provides a highly secure automated solution to help perform replication in just a few clicks using its interactive UI.
Want to take Hevo for a spin? Sign Up for a 14-day free trial and experience the feature-rich Hevo suite first hand.
Here are a few more blogs on MongoDB which might interest you: How to install MongoDB on Ubuntu and Top MongoDB ETL Challenges.
FAQ
1. Can I use MongoDB with NodeJS?
Yes you can use MongoDB with NodeJS.
2. How to connect MongoDB with Node.js 2025?
Run the following command npm install mongodb.
Import the dependencies create a client instance.
Connect to a Database and use the database.
3. How to connect to local MongoDB database in node JS?
Ensure MongoDB is running locally.
Use mongodb://localhost:27017
as the connection string:javascript
Replace "your_local_db_name"
with your database name, and ensure MongoDB is running on port 27017
(default).